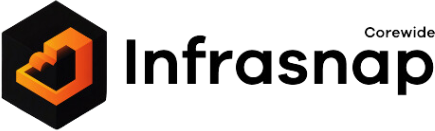
The module creates Azure Virtual Network with flexible number of subnets, and optionally creates Azure Bastion in the managed Virtual Network.
bash│
│ The "count" value depends on resource attributes that cannot be determined until apply, so Terraform cannot predict how many instances will be created. To work around this, use the -target argument to
│ first apply only the resources that the count depends on.
|
hclmodule "database" {
...
subnet_id = module.vnet.subnets["test"].id # breaks planning due to this value being unknown until apply
...
}
module "database" {
...
subnet_id = module.vnet.constructed_ids.subnets["test"] # does not break plan/apply, the value is predetermined
depends_on = [module.vnet] # ensures subnet ID will be returned only after the subnets are created
}
Once you have a Corewide Solutions Portal account, this one-time action will use your browser session to retrieve credentials:
shellterraform login solutions.corewide.com
Initialize mandatory providers:
Copy and paste into your Terraform configuration and insert the variables:
hclmodule "tf_azure_vnet" {
source = "solutions.corewide.com/azure/tf-azure-vnet/azurerm"
version = "~> 3.1.0"
# specify module inputs here or try one of the examples below
...
}
Initialize the setup:
shellterraform init
Corewide DevOps team strictly follows Semantic Versioning
Specification
to
provide our clients with products that have predictable upgrades between versions. We
recommend
pinning
patch versions of our modules using pessimistic
constraint operator (~>
) to prevent breaking changes during upgrades.
To get new features during the upgrades (without breaking compatibility), use
~> 3.1
and run
terraform init -upgrade
For the safest setup, use strict pinning with version = "3.1.0"
All notable changes to this project are documented here.
The format is based on Keep a Changelog, and this project adheres to Semantic Versioning.
constructed_ids
output with predetermined IDs of the managed resources in accordance with Azure fully qualified resource ID pattern conventionsubnets
variable validation rule to allow passing more than one correct service endpoint for a single subnetazurerm
provider version to 4.0
(Last version compatible with Terraform AzureRM v3)
routes
parameter to subnets
variable to manage routes for subnets' route tablesBREAKING CHANGE: now all the managed resources follow Azure naming conventions and resource names aren't compatible with previous module versions. Upgrading to this version will result in a complete re-creation of the Virtual Network resources. For more details see Upgrade Notes section
name_prefix
variable to name_suffix
v1.x
to v2.x
Since v2.0.0
, the module changes naming model of managed resources in accordance with Azure naming conventions, so all resources of the module will be re-created. Also, there is re-named variable name_prefix
to name_suffix
, it must be updated in module's declaration.
v2.x
to v3.x
Module from v3.0
has changed Azure provider version which isn't compatible with an old version. After the module version is upgraded, re-init module to upgrade Azure provider version.
Upgrade Azure provider version on project level to ~> 4.0:
hclterraform {
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "~> 4.0"
}
}
}
Upgrade project dependencies:
bashterraform init --upgrade
Create Virtual Network only:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "vnet" {
source = "solutions.corewide.com/azure/tf-azure-vnet/azurerm"
version = "~> 3.1"
name_suffix = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
address_space = [
"10.0.0.0/16",
]
tags = {
Project = "foo"
}
}
Create Virtual Network with 1 Subnet and Bastion Service:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "vnet" {
source = "solutions.corewide.com/azure/tf-azure-vnet/azurerm"
version = "~> 3.1"
name_suffix = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
bastion_pool = "10.0.250.0/26"
address_space = [
"10.0.0.0/16",
]
subnets = [
{
name_suffix = "baz"
addresses = [
"10.0.1.0/26",
]
},
]
tags = {
Project = "foo"
}
}
Create Virtual Network with one subnet and custom routes for its route table:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "vnet" {
source = "solutions.corewide.com/azure/tf-azure-vnet/azurerm"
version = "~> 3.1"
name_suffix = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
address_space = [
"10.0.0.0/16",
]
subnets = [
{
name_suffix = "baz"
addresses = [
"10.0.1.0/24",
]
routes = {
to-firewall = {
address_prefix = "0.0.0.0/0"
next_hop_in_ip_address = "178.10.23.23"
}
}
},
]
tags = {
Project = "foo"
}
}
Create Virtual Network with 2 Subnets packed in different configuration flavors:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "vnet" {
source = "solutions.corewide.com/azure/tf-azure-vnet/azurerm"
version = "~> 3.1"
name_suffix = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
address_space = [
"10.0.0.0/16",
]
subnets = [
{
name_suffix = "baz"
addresses = [
"10.0.1.0/24",
]
},
{
name_suffix = "biz"
addresses = [
"10.0.2.0/24",
]
service_endpoints = [
"Microsoft.AzureCosmosDB",
]
},
{
name_suffix = "qux"
delegation = "Microsoft.AzureCosmosDB/clusters"
addresses = [
"10.0.3.0/24",
]
},
]
tags = {
Project = "foo"
}
}
Variable | Description | Type | Default | Required | Sensitive |
---|---|---|---|---|---|
address_space |
The list of IP pools for Virtual Network | list(string) |
yes | no | |
name_suffix |
Naming suffix for the resources created by the module | string |
yes | no | |
region |
Resource Group location where Virtual Network will be created | string |
yes | no | |
resource_group_name |
The Resource Group Name in which resources should be created | string |
yes | no | |
bastion_pool |
Create Azure Bastion within the VN if set. Will create a special Subnet with provisioned IP pool to setup Bastion service (at least /26 mask required) | string |
no | no | |
subnets |
A list of Virtual Network Subnets to create | list(object) |
[] |
no | no |
subnets[*].addresses |
List of IP Pools to assign to the Subnet (within the VN pools) | list(string) |
yes | no | |
subnets[*].delegation |
If set, will delegate this Subnet to the Service defined here | string |
no | no | |
subnets[*].enable_private_endpoints |
Enable or Disable network policies for the private link endpoint on the Subnet. Set to true if using Private Link Endpoints |
bool |
false |
no | no |
subnets[*].name_suffix |
The name suffix of the Subnet to create | string |
yes | no | |
subnets[*].routes |
Routes to assign to subnet's route table. If not set, Azure defaults will be provisioned | map(object) |
{} |
no | no |
subnets[*].routes[<key>] |
Name of the route | string |
yes | no | |
subnets[*].routes[<key>].address_prefix |
The destination (CIDR or Azure Service Tag) to which the route applies | string |
yes | no | |
subnets[*].routes[<key>].next_hop_in_ip_address |
Contains the IP address packets should be forwarded to | string |
yes | no | |
subnets[*].service_endpoints |
If set, will allow the setup of listed Services endpoints within this Subnet for secure private communications. Additional configurations on the Service resources required | list(string) |
[] |
no | no |
tags |
A map of tags to apply (where key is a tag name and value is a tag value) | map(any) |
{} |
no | no |
Output | Description | Type | Sensitive |
---|---|---|---|
bastion |
Contains attributes of azurerm_bastion_host.bastion resource | computed |
no |
constructed_ids |
IDs of managed resources constructed in accordance with Azure fully qualified resource ID pattern convention. Available attributes are: vnet , subnets[*] , route_tables[*] , network_security_groups[*] , and bastion |
computed |
no |
network_security_groups |
The list of Network Security Groups associated to created Subnets. Passes attributes of azurerm_network_security_group.nsg resources | computed |
no |
route_tables |
The list of Route Tables associated to created Subnets. Passes attributes of azurerm_route_table.rt resources | computed |
no |
subnets |
The list of created Subnets within the Virtual Network. Passes attributes of azurerm_subnet.snet resources | computed |
no |
virtual_network |
Contains attributes of azurerm_virtual_network resource | resource |
no |
Dependency | Version | Kind |
---|---|---|
terraform |
>= 1.3 |
CLI |
hashicorp/azurerm |
~> 4.0 |
provider |