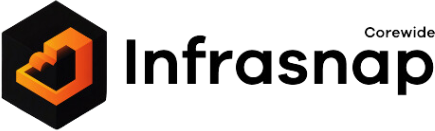
The module manages an Azure Container Registry (creates a new one or manages an existing one), and optionally provides role-assigned registry authorization to Azure resources based on their Principal ID, i.e. Azure Kubernetes Service cluster, Container Instances, managed Virtual Machines, etc.
The module can optionally manage images retention by means of Azure ACR Tasks for images purge, which will purge images in the entire registry based on:
Once you have a Corewide Solutions Portal account, this one-time action will use your browser session to retrieve credentials:
shellterraform login solutions.corewide.com
Initialize mandatory providers:
Copy and paste into your Terraform configuration and insert the variables:
hclmodule "tf_azure_acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0.0"
# specify module inputs here or try one of the examples below
...
}
Initialize the setup:
shellterraform init
Corewide DevOps team strictly follows Semantic Versioning
Specification
to
provide our clients with products that have predictable upgrades between versions. We
recommend
pinning
patch versions of our modules using pessimistic
constraint operator (~>
) to prevent breaking changes during upgrades.
To get new features during the upgrades (without breaking compatibility), use
~> 2.0
and run
terraform init -upgrade
For the safest setup, use strict pinning with version = "2.0.0"
All notable changes to this project are documented here.
The format is based on Keep a Changelog, and this project adheres to Semantic Versioning.
azurerm
provider's version to 4.2
(Last version compatible with Terraform AzureRM v3)
v1.x
to v2.x
Module from v2.0
has changed AzureRM provider version which isn't compatible with an old version. After the module version is upgraded, re-init module to upgrade AzureRM provider version.
Upgrade AzureRM providers version on project level to ~> 4.2:
hclrequired_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "~> 4.2"
}
}
Upgrade project dependencies:
bashterraform init --upgrade
Create only Container Registry:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0"
name = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
}
Create container registry with a default images retention settings by all of the included repositories:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0"
name = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
images_retention = [
{
name_suffix = "all"
},
]
}
Create container registry with a custom images retention settings:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0"
name = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
images_retention = [
{
name_suffix = "web-applications" # results in a task name `images-retention-web-applications`
cron = "*/30 * * * *" # run task every 30 minutes
duration = "12h" # delete all images that were last modified 12 hours ago or more
filters = ["hello-world:^pre-release.*", "web-app:.*"] # matches tags beginning with `pre-release` in the `hello-world` repository only and all tags in the `web-app` repository
keep_images = 10 # keep the latest 10 images of to-be-deleted tags
},
{
name_suffix = "backend" # results in a task name `images-retention-backend`
cron = "0 */12 * * *" # run task every 12 hours
duration = "14d12h" # delete all images that were last modified 14 days and 12 hours ago or more
filters = ["backend:^release-channel.*"] # matches tags beginning with `release-channel` in the `backend` repository only
keep_images = 20 # keep the latest 20 images of to-be-deleted tags
},
]
}
Create a Container Registry and grant access to managed Kubernetes cluster with read-only permissions:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0"
name = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
authorize_access = [
{
identity = azurerm_kubernetes_cluster.biz.kubelet_identity[0].object_id
},
]
}
Setup for Container Registry management with granting access to several managed Kubernetes clusters in separate workspaces:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0"
name = "bar"
create = terraform.workspace == "dev"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
authorize_access = [
{
identity = azurerm_kubernetes_cluster.biz.kubelet_identity[0].object_id
permissions = terraform.workspace == "dev" ? "rw" : "ro"
},
]
}
Setup for Container Registry management with granting access to managed Kubernetes cluster, Virtual Machine and Container Group:
hclresource "azurerm_resource_group" "foo" {
name = "foo"
location = "eastus"
}
module "acr" {
source = "solutions.corewide.com/azure/tf-azure-acr/azurerm"
version = "~> 2.0"
name = "bar"
resource_group_name = azurerm_resource_group.foo.name
region = azurerm_resource_group.foo.location
authorize_access = [
# Authorize AKS cluster with a read-only permissions
{
identity = azurerm_kubernetes_cluster.biz.kubelet_identity[0].object_id
},
# Authorize Container Group with a read-only permissions
{
identity = azurerm_container_group.baz.identity[0].principal_id
},
# Authorize VM of managed CI plathorm with read-write permissions
{
identity = azurerm_virtual_machine.ci.identity[0].principal_id
permissions = "rw"
}
]
}
Variable | Description | Type | Default | Required | Sensitive |
---|---|---|---|---|---|
name |
The name of the Container Registry | string |
yes | no | |
region |
Resource Group location where the Container Registry will be created | string |
yes | no | |
resource_group_name |
The Resource Group Name in which resources will be created | string |
yes | no | |
authorize_access |
Parameters for granting role-assigned registry authorization to Container Registry | list(object) |
[] |
no | no |
authorize_access[*].identity |
Defines an ID of Azure resource identity which should have an access to managed Container Registry (Must be specified if integration with container registry is required) | string |
yes | no | |
authorize_access[*].permissions |
Defines the access level of Azure resource to Container Registry (ro (Read-only) or rw (Read-write)) |
string |
ro |
no | no |
create |
Enable/disable Container Registry creation | bool |
true |
no | no |
images_retention |
A list of images retention settings | list(object) |
[] |
no | no |
images_retention[*].cron |
The cron expression for the retention schedule | string |
0 0 * * * |
no | no |
images_retention[*].duration |
A Go-style duration string to indicate a duration beyond which images are deleted. The duration consists of a sequence of one or more decimal numbers, each with a unit suffix. Valid time units include "d" for days, "h" for hours, and "m" for minutes. For example, --ago 2d3h6m selects all filtered images last modified more than two days, 3 hours, and 6 minutes ago, and --ago 1.5h selects images last modified more than 1.5 hours ago |
string |
30d |
no | no |
images_retention[*].filters |
A list of repository name regular expression and a tag name regular expression to filter images in the registry | list(string) |
['.*:.*'] |
no | no |
images_retention[*].keep_images |
Number of latest to-be-deleted tags to keep, at least x number of latest tags that could be deleted meeting all other filter criteria | number |
50 |
no | no |
images_retention[*].name_suffix |
The name suffix of the ACR task adding to images-retention-<name_suffix> name |
string |
yes | no | |
sku |
The SKU (purchasable Stock Keeping Unit) name of the Container Registry (Basic , Standard or Premium ) |
string |
Basic |
no | no |
Output | Description | Type | Sensitive |
---|---|---|---|
registry |
Contains a set of the Container Registry attributes | computed |
no |
Dependency | Version | Kind |
---|---|---|
terraform |
>= 1.3 |
CLI |
hashicorp/azurerm |
~> 4.2 |
provider |